寫了一個圖片Resize的工具,但是後來發現如果用nginx好像根本不用寫…XDD
不過既然寫了就還是紀錄一下吧…也許未來哪天有機會用到
設定縮放或裁切的方式 - EnumModels.CutType
1 | public class EnumModels |
主要邏輯 - ImageHelper
- ResizeImage 縮放或裁切
- BitmapToByte
1 | public class ImageHelper |
.Net Core WebApi
- OnPostUploadAsync: 上傳圖片存放至
/wwwroot/UploadImage
下 - GetAll: 取得所有
/wwwroot/UploadImage
內的圖片 - ResizeImage:
- [Route(“w/{width}/{name}”)] : 固定寬等比例縮放
- [Route(“h/{height}/{name}”)] : 固定高等比例縮放
- [Route(“wh/{width}x{height}/{name}”)] : 固定寬高切中間
- MemoryCache: 避免重複使用浪費時間,記得在
Startup.cs
加上services.AddMemoryCache();
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83[ ]
[ ]
public class ImageController : ControllerBase
{
private readonly IHostingEnvironment _hostingEnvironment;
private readonly IMemoryCache _memoryCache;
private static string IMAGE_UPLOAD_CURRENT_PATH;
public ImageController (IHostingEnvironment hostingEnvironment, IMemoryCache memoryCache)
{
_hostingEnvironment = hostingEnvironment;
_memoryCache = memoryCache;
if (IMAGE_UPLOAD_CURRENT_PATH == null)
{
IMAGE_UPLOAD_CURRENT_PATH = Path.Combine(_hostingEnvironment.ContentRootPath, "wwwroot", "UploadImage");
if (System.IO.Directory.Exists(IMAGE_UPLOAD_CURRENT_PATH))
System.IO.Directory.CreateDirectory(IMAGE_UPLOAD_CURRENT_PATH);
}
}
[ ]
public async Task<IActionResult> OnPostUploadAsync([FromForm(Name = "image")]List<IFormFile> files)
{
long size = files.Sum(f => f.Length);
foreach (var formFile in files)
{
if (formFile.Length > 0)
{
var filePath = Path.Combine(IMAGE_UPLOAD_CURRENT_PATH, formFile.FileName);
if (System.IO.File.Exists(filePath))
{
return BadRequest($"檔案已存在, { formFile.FileName }");
}
using (var stream = System.IO.File.Create(filePath))
{
await formFile.CopyToAsync(stream);
}
}
}
return Ok(new { count = files.Count, size });
}
[ ]
public IActionResult GetAll()
{
var imgFiles = _memoryCache.Get(CacheKey.UPLOAD_IMAGE_FILES) as IEnumerable<string>;
if (imgFiles == null)
{
imgFiles = Directory.GetFiles(IMAGE_UPLOAD_CURRENT_PATH).Select(x => Path.GetFileName(x));
_memoryCache.Set(CacheKey.UPLOAD_IMAGE_FILES, imgFiles);
}
return Ok(new {
code = 20000,
data = imgFiles
});
}
[ ]
[ ]
[ ]
[ ]
public IActionResult GetResizeImage(int width, int height, string name, int cutType = 0)
{
try
{
var imgPath = Path.Combine(IMAGE_UPLOAD_CURRENT_PATH, name);
var imgType = Path.GetExtension(name).Replace(".", "").ToLower();
var contentTypeStr = ImageType.GetTypeByFileExtension(imgType);
//圖片不存在
if (!new FileInfo(imgPath).Exists)
throw new Exception("圖片不存在");
var bitmap = ImageHelper.ResizeImage(imgPath, (EnumModels.CutType)cutType, width, height);
return new FileContentResult(ImageHelper.BitmapToByte(bitmap), contentTypeStr);
}
catch //(Exception ex)
{
return NotFound();
}
}
}
最後,如果你有興趣學程式,可以參考下列平台:
裡面有很多豐富的課程,線上學習,不用出門唷!
↓↓↓ 如果喜歡我的文章,可以幫我按個Like! ↓↓↓
>> 或者,請我喝杯咖啡,這樣我會更有動力唷! <<<
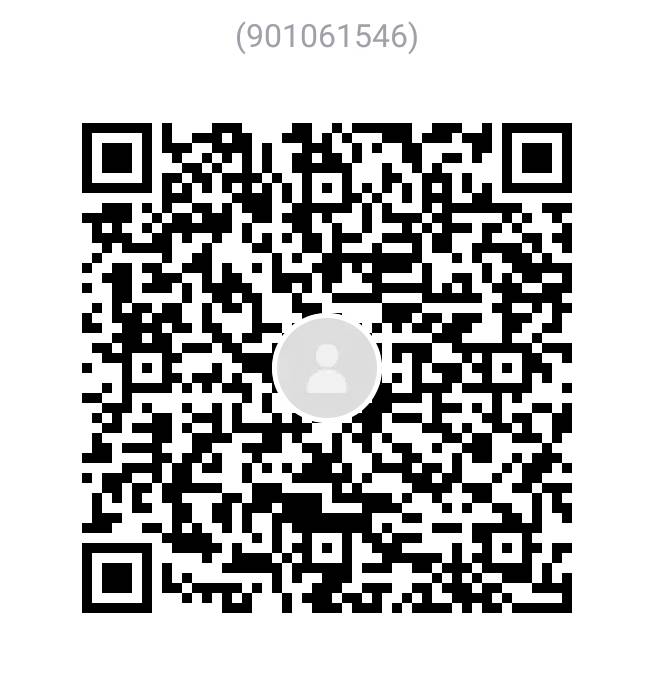
街口支付
街口帳號: 901061546